For my current Amiga project, I had a little prototyping chain like this:
- Write a code module in JavaScript (table generator, display code, etc.)
- Use <canvas> & co. for visualization
- Use data structures similar to the target
- Port the working prototype to 68k assembly
- Test, debug, sprinkle in some logging, debug more, get angry, etc.
The turnaround times with an HTML prototype are nice, but the disconnect of prototype code and real code sucks. Why not write in 68k assembly from the start? With the help of a 68000 CPU emulator, I tried this:
- Write a code module in 68k assembly
- Write a simulation shim with a custom visualization or data table
- Assemble, simulate, update visualization
Writing a custom code tester for each module seems convoluted, but the turnaround times are instant, and there is no need to write the code twice. Invoking vasm when you press CTRL+S is really fast, directly running the assembled binary as well. Here’s a texture generator in action:
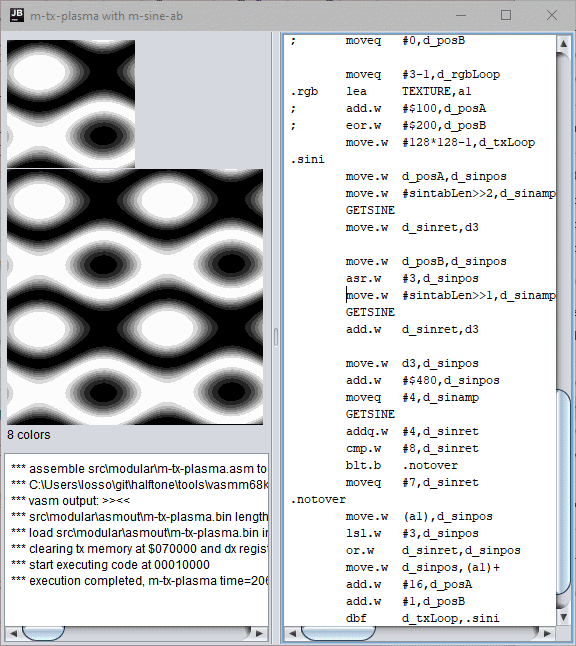
Running a code simulation is as easy as:
// run "vasmm68k_mot -quiet -Fbin -o tx.bin tx.asm", then: val STACK = 0x00800 val CODE = 0x10000 val SINTAB = 0x60000 val TEXTURE = 0x70000 val mem = MemorySpace(512) loadFileIntoMemory(File("sintab.bin"), mem, SINTAB) loadFileIntoMemory(File("tx.bin"), mem, CODE) val cpu = MC68000() cpu.setAddressSpace(mem) cpu.setAddrRegisterLong(0, TEXTURE) cpu.setAddrRegisterLong(7, STACK) cpu.reset() cpu.pc = CODE val addressAfterCode = CODE + File("tx.bin").length() while (cpu.pc < addressAfterCode) { cpu.execute() }
Of course writing the prototyping and design tools natively as well would be even cooler… But so far I’m quite happy, I quickly found some subtle irregularities, and texture code testing was a breeze.
Shout-out to Tony Headford for m68k!