In terms of debugging, I’m a printer, not a stepper. Yes, single-stepping and breakpoints are nice, but I like this:
PUTMSG 10,<"searching for ""%s""">,a0 ... move.l next_part(a6),a0 PUTMSG 10,<"will jsr to %p">,a0 jsr (a0) PUTMSG 10,<"returned with $%lx = %ld">,d0,d0
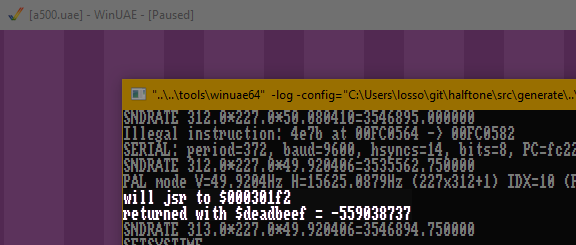
The inner debugging monologue becomes so much more relaxing and constructive when strategic log statements are providing the answers.
- WTF, where does this pointer come from?
- Well, you overwrote the lower word, apparently.
- How in hell are those sine values all off?!
- I’d say
$0000
looks like a weird amplitude. - I swear to god, my memory allocator cannot possibly fail here!
- It can and it does, especially when you try to reserve a negative value.
You get the idea. :) The following trick is old news, by the way, but not exactly well-documented, either.
The hard way
In the past, I’ve been using a logging setup like this:
- Configure WinUAE to expose the serial port via TCP on port 1234
- Do the log message formatting in assembly, with the help of exec.library’s RawDoFmt (which still works when you’ve taken over the system)
- Send out the formatted log output through the serial port, manually banging SERDAT and SERPER
- Display the live log with
netcat
or a custom tool that connects to WinUAE’s serial TCP port
A rather convoluted process – but there’s an easier way!
(It does have the advantage that you could also debug on real hardware this way – just connect the Amiga’s serial port to a terminal! I have used that exactly once and it was cool and all, but also cumbersome.)
Easy and light-weight with WinUAE
Ever since release 4.4.0, WinUAE has included a more elegant “API” to do printf calls from your Amiga code. (I’ve learned about this when I started using the PLatOS demo framework for new art – it comes with a million amenities and also has a logging macro built in.)
- Start WinUAE with
-log -s debug_mem=true
- Write your printf arguments to
$bfff00
one after another - Put a pointer to your format string into
$bfff04
- Done! WinUAE will do the formatting for you and display your message in its console/debug window
Neat, huh? Here’s a self-contained snippet for a “PUTMSG” macro, modelled after its PLatOS counterpart (but only supporting seven arguments for compatibility):
- debug-putmsg.i
Self-contained PUTMSG macro for formatted log messages in WinUAE
In your code, do this:
DEBUG_DETAIL = 10 ; 0 disables logging include debug-putmsg.i PUTMSG 10,<"Stack at %p, d0.w is %x">,a7,d0 PUTMSG 8,<"Low-level message"> ; not printed: 8 < 10
Format | Output |
---|---|
%d | Decimal word |
%ld | Decimal longword |
%x | Hex word |
%lx | Hex longword |
%c | Byte as char |
%s | String (null-terminated, passed as pointer) |
%p | Zero-padded hex longword, 8 hex digits with $ prefix |
%08lx | Zero-padded hex longword, 8 hex digits |
The macro passes all arguments as longwords. If you pass a word from a memory address, you need to subtract 2:
PUTMSG 10,<"ticks = %d">,ticks-2(a6)